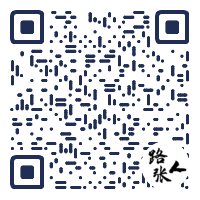
扫码关注微信公众号
回复“面试手册”,获取本站PDF版
回复“简历”,获取高质量简历模板
回复“加群”,加入程序员交流群
回复“电子书”,获取程序员类电子书
题目描述
输入一个链表的头节点,从尾到头反过来返回每个节点的值(用数组返回)。
示例 1:
输入:head = [1,3,2]
输出:[2,3,1]
限制:
0 <= 链表长度 <= 10000
题解 1
(迭代) O(n)
从前往后遍历链表,存储每个节点的值到答案数组中,然后反转答案数组就是从尾到头打印链表的结果。
时间复杂度
O(n)
空间复杂度
O(n)
C++ 代码
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
vector<int> reversePrint(ListNode* head) {
vector<int> res;
for (auto p = head; p; p = p->next) res.push_back(p->val);
reverse(res.begin(), res.end());
return res;
}
};
Java 代码
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode(int x) { val = x; }
* }
*/
class Solution {
public int[] reversePrint(ListNode head) {
List<Integer> resList = new ArrayList<>();
ListNode p = head;
while (p != null) {
resList.add(p.val);
p = p.next;
}
Collections.reverse(resList);
int[] res = new int[resList.size()];
for (int i = 0; i < resList.size(); i ++ ) {
res[i] = resList.get(i);
}
return res;
}
}
Python 代码
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, x):
# self.val = x
# self.next = None
class Solution:
def reversePrint(self, head: ListNode) -> List[int]:
res_list = []
p = head
while p:
res_list.append(p.val)
p = p.next
return res_list[::-1]
题解 2
(递归) O(n)
递归的出口条件:当前节点为空,返回空数组。 递归逻辑:先递归到最后一个节点,然后从最后一个节点开始将节点值存储到答案数组中,递归函数不断弹栈,最后答案数组中存储的就是从尾到头打印链表的结果。
时间复杂度
O(n)
空间复杂度
存储答案的空间 O(n),包含递归系统栈所需的空间 O(n)。
C++ 代码
/**
* Definition for singly-linked list.
* struct ListNode {
* int val;
* ListNode *next;
* ListNode(int x) : val(x), next(NULL) {}
* };
*/
class Solution {
public:
vector<int> res;
vector<int> reversePrint(ListNode* head) {
if (!head) return {};
reversePrint(head->next);
res.push_back(head->val);
return res;
}
};
Java 代码
/**
* Definition for singly-linked list.
* public class ListNode {
* int val;
* ListNode next;
* ListNode(int x) { val = x; }
* }
*/
class Solution {
List<Integer> res = new ArrayList<>();
public int[] reversePrint(ListNode head) {
reverseList(head);
int[] result = new int[res.size()];
for (int i = 0; i < res.size(); i ++ ) {
result[i] = res.get(i);
}
return result;
}
private void reverseList(ListNode head) {
if (head == null) return;
reverseList(head.next);
res.add(head.val);
}
}
Python 代码
# Definition for singly-linked list.
# class ListNode:
# def __init__(self, x):
# self.val = x
# self.next = None
class Solution:
def reversePrint(self, head: ListNode) -> List[int]:
self.res = []
def reverseList(node):
if not node:
return
reverseList(node.next)
self.res.append(node.val)
reverseList(head)
return self.res
本文由读者提供,Github地址:https://github.com/tonngw
点击面试手册,获取本站面试手册PDF完整版