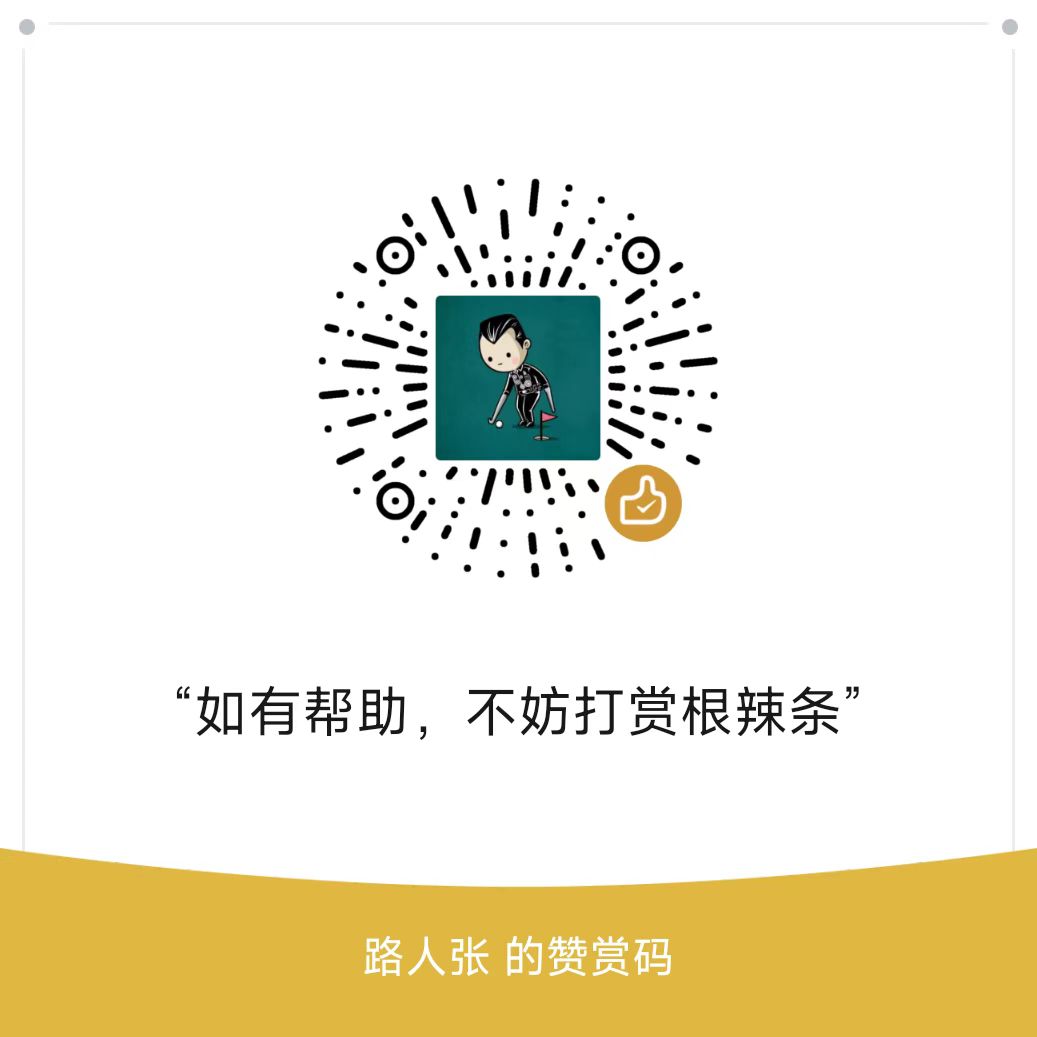
网站救助计划
1.为阅读体验,本站无任何广告,也无任何盈利方法,站长一直在用爱发电,现濒临倒闭,希望有能力的同学能帮忙分担服务器成本
2.捐助10元及以上同学,可添加站长微信lurenzhang888,备注捐助,网站倒闭后可联系站长领取本站pdf内容
3.若网站能存活下来,后续将会持续更新内容
题目描述
输入一个矩阵,按照从外向里以顺时针的顺序依次打印出每一个数字。
示例 1:
输入:matrix = [[1,2,3],[4,5,6],[7,8,9]]
输出:[1,2,3,6,9,8,7,4,5]
示例 2:
输入:matrix = [[1,2,3,4],[5,6,7,8],[9,10,11,12]]
输出:[1,2,3,4,8,12,11,10,9,5,6,7]
限制:
- 0 <= matrix.length <= 100
- 0 <= matrix[i].length <= 100
题解
(模拟) O(n * m)
和 螺旋矩阵II 思路一样。使用两个数组定义上右下左四个方向以及方向变量,开始默认从右走,用一个二维 bool 数组 st 存储每个位置是否被访问过,如果当前位置没有越界且没有被访问过就将其加入到答案数组中,否则改变方向继续往前走,直到走完矩阵中的所有元素。
扩展题目:59. 螺旋矩阵 II
时间复杂度
O(n * m)
空间复杂度
O(n^2)
C++ 代码
class Solution {
public:
vector<int> spiralOrder(vector<vector<int>>& matrix) {
vector<int> res;
if (matrix.empty() || matrix[0].empty()) return res;
int n = matrix.size(), m = matrix[0].size();
vector<vector<bool>> st(n, vector<bool>(m, false));
int dx[4] = {-1, 0, 1, 0}, dy[4] = {0, 1, 0, -1};
for (int x = 0, y = 0, t = 0, d = 1; t < n * m; t ++ ) {
res.push_back(matrix[x][y]);
st[x][y] = true;
int a = x + dx[d], b = y + dy[d];
if (a < 0 || a >= n || b < 0 || b >= m || st[a][b]) {
d = (d + 1) % 4;
a = x + dx[d], b = y + dy[d];
}
x = a, y = b;
}
return res;
}
};
Java 代码
class Solution {
public int[] spiralOrder(int[][] matrix) {
if (matrix == null || matrix.length == 0 || matrix[0].length == 0) {
return new int[0];
}
int n = matrix.length, m = matrix[0].length;
int[] res = new int[n * m];
boolean[][] st = new boolean[n][m];
int[] dx = {-1, 0, 1, 0}, dy = {0, 1, 0, -1};
int x = 0, y = 0, t = 0, d = 1;
while (t < n * m) {
res[t] = matrix[x][y];
st[x][y] = true;
int a = x + dx[d], b = y + dy[d];
if (a < 0 || a >= n || b < 0 || b >= m || st[a][b]) {
d = (d + 1) % 4;
a = x + dx[d];
b = y + dy[d];
}
x = a;
y = b;
t ++ ;
}
return res;
}
}
Python 代码
class Solution:
def spiralOrder(self, matrix: List[List[int]]) -> List[int]:
res = []
if not matrix or not matrix[0]:
return res
n = len(matrix)
m = len(matrix[0])
st = [[False] * m for _ in range(n)]
dx = [-1, 0, 1, 0]
dy = [0, 1, 0, -1]
x = 0
y = 0
d = 1
for t in range(n * m):
res.append(matrix[x][y])
st[x][y] = True
a = x + dx[d]
b = y + dy[d]
if a < 0 or a >= n or b < 0 or b >= m or st[a][b]:
d = (d + 1) % 4
a = x + dx[d]
b = y + dy[d]
x = a
y = b
return res
本文由读者提供,Github地址:https://github.com/tonngw
点击面试手册,获取本站面试手册PDF完整版