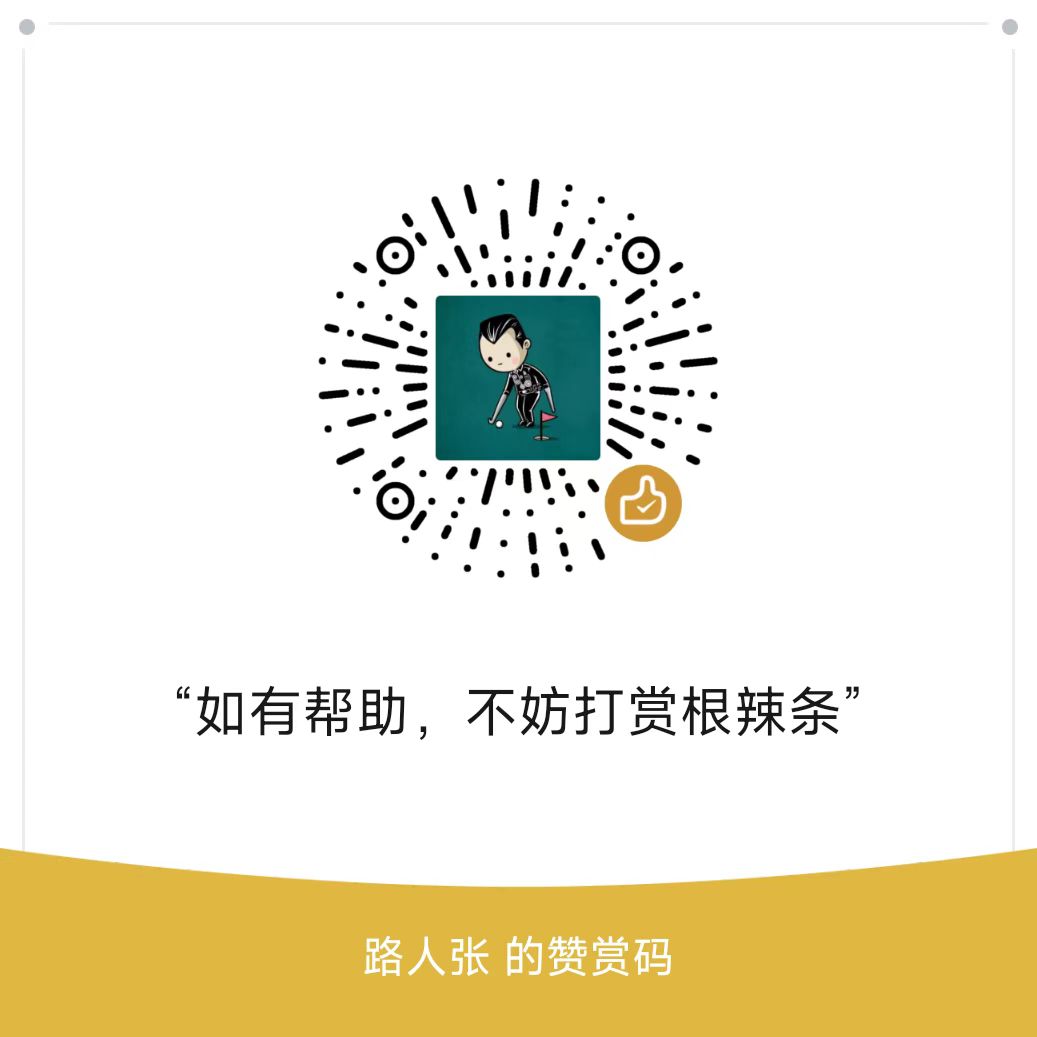
网站救助计划
1.为阅读体验,本站无任何广告,也无任何盈利方法,站长一直在用爱发电,现濒临倒闭,希望有能力的同学能帮忙分担服务器成本
2.捐助10元及以上同学,可添加站长微信lurenzhang888,备注捐助,网站倒闭后可联系站长领取本站pdf内容
3.若网站能存活下来,后续将会持续更新内容
题目描述
输入一棵二叉树的根节点,求该树的深度。从根节点到叶节点依次经过的节点(含根、叶节点)形成树的一条路径,最长路径的长度为树的深度。
例如:
给定二叉树 [3,9,20,null,null,15,7],
3
/ \
9 20
/ \
15 7
返回它的最大深度 3 。
提示:
- 节点总数 <= 10000
题解 1
(BFS,迭代) O(n)
我们可以发现二叉树的最大深度正好是二叉树的层树,所以只需在层序遍历每一层的过程中记录层数即可,每多一层深度就加 1。
时间复杂度
二叉树中的每个节点只会被遍历一次,所以时间复杂度为 O(n)。
空间复杂度
O(logn),最坏情况下二叉树呈链状,空间复杂度为 O(n)
C++ 代码
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode() : val(0), left(nullptr), right(nullptr) {}
* TreeNode(int x) : val(x), left(nullptr), right(nullptr) {}
* TreeNode(int x, TreeNode *left, TreeNode *right) : val(x), left(left), right(right) {}
* };
*/
class Solution {
public:
int maxDepth(TreeNode* root) {
int res = 0;
if (!root) return res;
queue<TreeNode*> q;
q.push(root);
while (q.size()) {
int sz = q.size();
while (sz -- ) {
auto t = q.front();
q.pop();
if (t->left) q.push(t->left);
if (t->right) q.push(t->right);
}
res ++ ;
}
return res;
}
};
Java 代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public int maxDepth(TreeNode root) {
int res = 0;
if (root == null) return res;
Queue<TreeNode> q = new LinkedList<>();
q.offer(root);
while (!q.isEmpty()) {
int sz = q.size();
while (sz > 0) {
TreeNode t = q.poll();
if (t.left != null) q.offer(t.left);
if (t.right != null) q.offer(t.right);
sz -- ;
}
res ++ ;
}
return res;
}
}
Python 代码
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def maxDepth(self, root: TreeNode) -> int:
res = 0
if root is None:
return res
q = collections.deque()
q.append(root)
while q:
sz = len(q)
for _ in range(sz):
t = q.popleft()
if t.left:
q.append(t.left)
if t.right:
q.append(t.right)
res += 1
return res
题解 2
(DFS,递归) O(n)
- 递归函数的含义:求当前树的高度(高度即最大深度)
- 递归的出口:如果当前节点为空节点,高度为 0
- 单层递归的逻辑:按后序遍历的顺序,先求左子树的高度,再求右子树的高度,最后取左右子树高度的最大值 + 1 即为当前节点的高度。
时间复杂度
二叉树中的每个节点只会被遍历一次,所以时间复杂度为 O(n)。
空间复杂度
O(logn),最坏情况下二叉树呈链状,空间复杂度为 O(n)
C++ 代码
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
class Solution {
public:
int maxDepth(TreeNode* root) {
if (!root) return 0;
return max(maxDepth(root->left), maxDepth(root->right)) + 1;
}
};
Java 代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public int maxDepth(TreeNode root) {
if (root == null) return 0;
return Math.max(maxDepth(root.left), maxDepth(root.right)) + 1;
}
}
Python 代码
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def maxDepth(self, root: TreeNode) -> int:
if root is None:
return 0
return max(self.maxDepth(root.left), self.maxDepth(root.right)) + 1
本文由读者提供,Github地址:https://github.com/tonngw
点击面试手册,获取本站面试手册PDF完整版