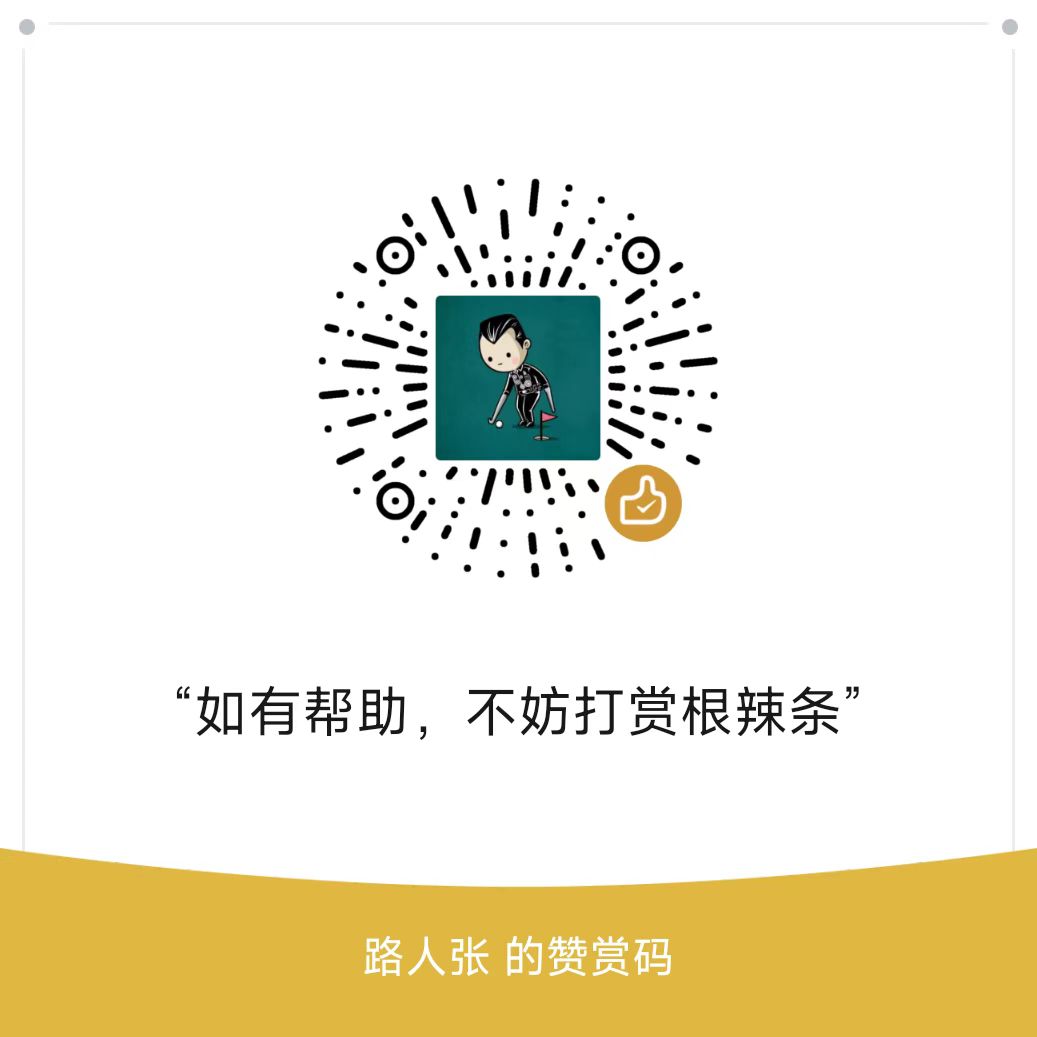
网站救助计划
1.为阅读体验,本站无任何广告,也无任何盈利方法,站长一直在用爱发电,现濒临倒闭,希望有能力的同学能帮忙分担服务器成本
2.捐助10元及以上同学,可添加站长微信lurenzhang888,备注捐助,网站倒闭后可联系站长领取本站pdf内容
3.若网站能存活下来,后续将会持续更新内容
题目描述
请完成一个函数,输入一个二叉树,该函数输出它的镜像。
例如输入:
4
/ \
2 7
/ \ / \
1 3 6 9
镜像输出:
4
/ \
7 2
/ \ / \
9 6 3 1
示例 1:
输入:root = [4,2,7,1,3,6,9]
输出:[4,7,2,9,6,3,1]
限制:
0 <= 节点个数 <= 1000
题解
(BFS,前序遍历) O(n)
翻转二叉树就是翻转二叉树的每个节点的左右孩子,把握住这一点,首先我们需要遍历二叉树,遍历二叉树的方法有很多,比如:前序遍历、后序遍历、层序遍历,但只有中序遍历不可以。
为什么中序边里不可以? 中序遍历会导致某些节点的左右孩子被翻转两次,而某些节点却一次都没有翻转,这是和中序遍历的过程有关系的,当我们遍历的当前节点是根节点的时候,首先翻转根节点的左右孩子,下一步要将根节点的右孩子的左边一条链都加入到栈中,而此时的右孩子恰恰是原树的左孩子,所以原树的左子树又会被翻转一次,而原树的右孩子却一次都没有被翻转,因此不能用中序遍历来翻转二叉树。
时间复杂度
O(n)
空间复杂度
O(logn),最坏情况下二叉树呈链状,空间复杂度为 O(n)。
C++代码
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
class Solution {
public:
TreeNode* mirrorTree(TreeNode* root) {
if (!root) return nullptr;
mirrorTree(root->left);
mirrorTree(root->right);
swap(root->left, root->right);
return root;
}
};
Java 代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public TreeNode mirrorTree(TreeNode root) {
if (root == null) {
return null;
}
mirrorTree(root.left);
mirrorTree(root.right);
TreeNode temp = root.left;
root.left = root.right;
root.right = temp;
return root;
}
}
Python 代码
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def mirrorTree(self, root: TreeNode) -> TreeNode:
if not root:
return None
self.mirrorTree(root.left)
self.mirrorTree(root.right)
root.left, root.right = root.right, root.left
return root
本文由读者提供,Github地址:https://github.com/tonngw
点击面试手册,获取本站面试手册PDF完整版