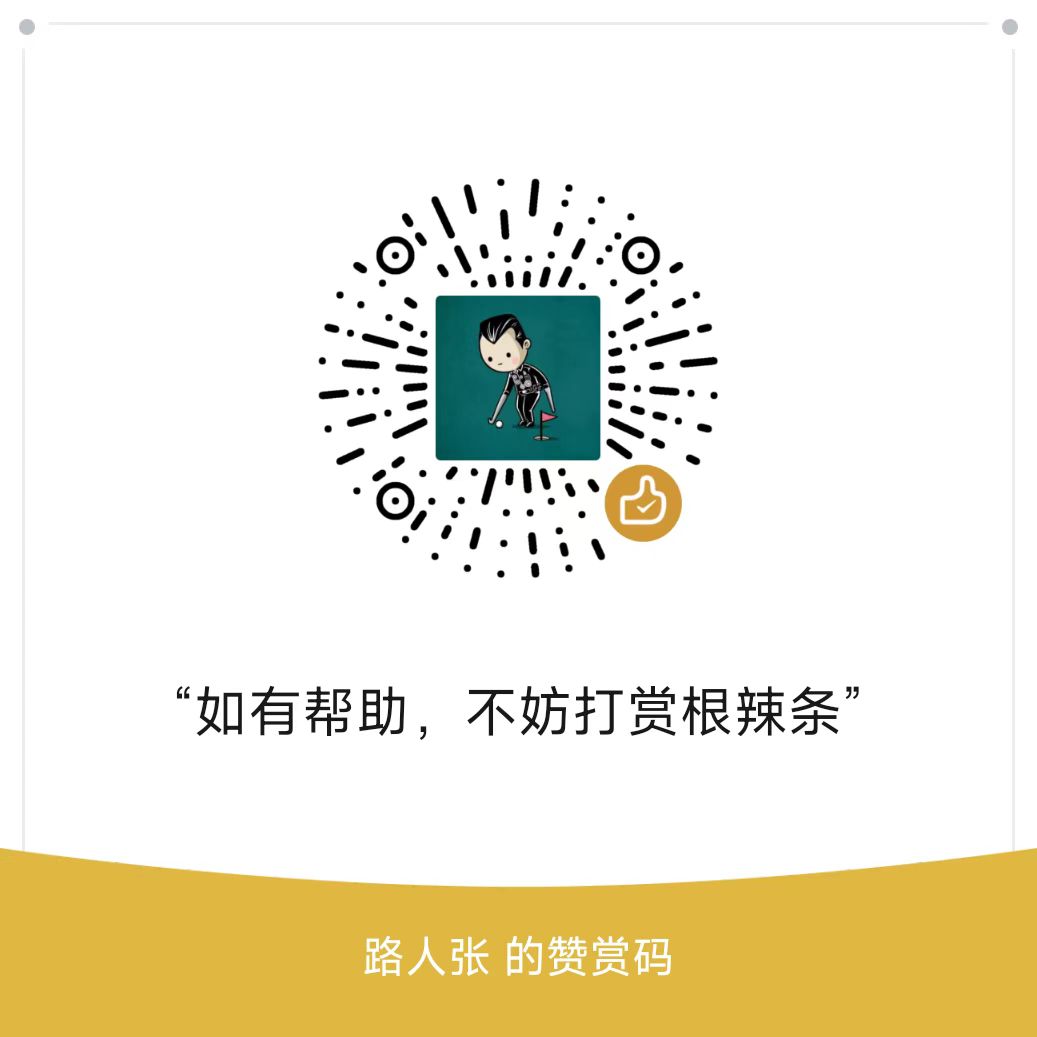
网站救助计划
1.为阅读体验,本站无任何广告,也无任何盈利方法,站长一直在用爱发电,现濒临倒闭,希望有能力的同学能帮忙分担服务器成本
2.捐助10元及以上同学,可添加站长微信lurenzhang888,备注捐助,网站倒闭后可联系站长领取本站pdf内容
3.若网站能存活下来,后续将会持续更新内容
题目描述
从上到下打印出二叉树的每个节点,同一层的节点按照从左到右的顺序打印。
例如: 给定二叉树: [3,9,20,null,null,15,7]
,
3
/ \
9 20
/ \
15 7
返回:
[3,9,20,15,7]
提示:
节点个数 <= 1000
题解
(BFS,层序遍历)) O(n)
使用宽度优先搜索配合队列层序遍历二叉树
- 首先先将根节点入队列
- 如果队列不为空,进行以下循环
- 每次弹出队头元素,并将其对应的值加入到答案,如果当前节点有左孩子,则将左孩子入队列,如果当前节点有右孩子,则将右孩子入队列,代表下一层节点。
时间复杂度
二叉树的每个节点只会被遍历一次,所以时间复杂度为 O(n)。
空间复杂度
存储答案需要额外 O(n^2) 的空间,队列需要额外 O(n) 的空间。
C++ 代码
/**
* Definition for a binary tree node.
* struct TreeNode {
* int val;
* TreeNode *left;
* TreeNode *right;
* TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
class Solution {
public:
vector<int> levelOrder(TreeNode* root) {
vector<int> res;
queue<TreeNode*> q;
if (root) q.push(root);
while (q.size()) {
auto t = q.front();
q.pop();
res.push_back(t->val);
if (t->left) q.push(t->left);
if (t->right) q.push(t->right);
}
return res;
}
};
Java 代码
/**
* Definition for a binary tree node.
* public class TreeNode {
* int val;
* TreeNode left;
* TreeNode right;
* TreeNode(int x) { val = x; }
* }
*/
class Solution {
public int[] levelOrder(TreeNode root) {
if (root == null) {
return new int[0];
}
List<Integer> resList = new ArrayList<>();
Queue<TreeNode> q = new LinkedList<>();
q.offer(root);
while (!q.isEmpty()) {
TreeNode t = q.poll();
resList.add(t.val);
if (t.left != null) {
q.offer(t.left);
}
if (t.right != null) {
q.offer(t.right);
}
}
int[] res = new int[resList.size()];
for (int i = 0; i < resList.size(); i ++ ) {
res[i] = resList.get(i);
}
return res;
}
}
Python 代码
# Definition for a binary tree node.
# class TreeNode:
# def __init__(self, x):
# self.val = x
# self.left = None
# self.right = None
class Solution:
def levelOrder(self, root: TreeNode) -> List[int]:
if not root:
return []
res = []
q = deque()
q.append(root)
while q:
t = q.popleft()
res.append(t.val)
if t.left:
q.append(t.left)
if t.right:
q.append(t.right)
return res
本文由读者提供,Github地址:https://github.com/tonngw
点击面试手册,获取本站面试手册PDF完整版